MinIO使用
MiniO 单机部署以及使用
[中文官方文档](MinIO Quickstart Guide| Minio中文文档)
1. docker-compose部署
version: '3'
services:
minio:
image: minio/minio
hostname: "minio"
ports:
- 9000:9000
- 9001:9001
environment:
MINIO_ROOT_USER: admin #管理后台用户名
MINIO_ROOT_PASSWORD: admin123321 #管理后台密码,最小8个字符
volumes:
- /Users/docker/minio/data:/data #映射当前目录下的data目录至容器内/data目录
- /Users/docker/minio/config:/root/.minio/ #映射配置目录
command: server --console-address ':9001' /data #指定容器中的目录 /data
privileged: true
restart: always
2.登陆管理页面
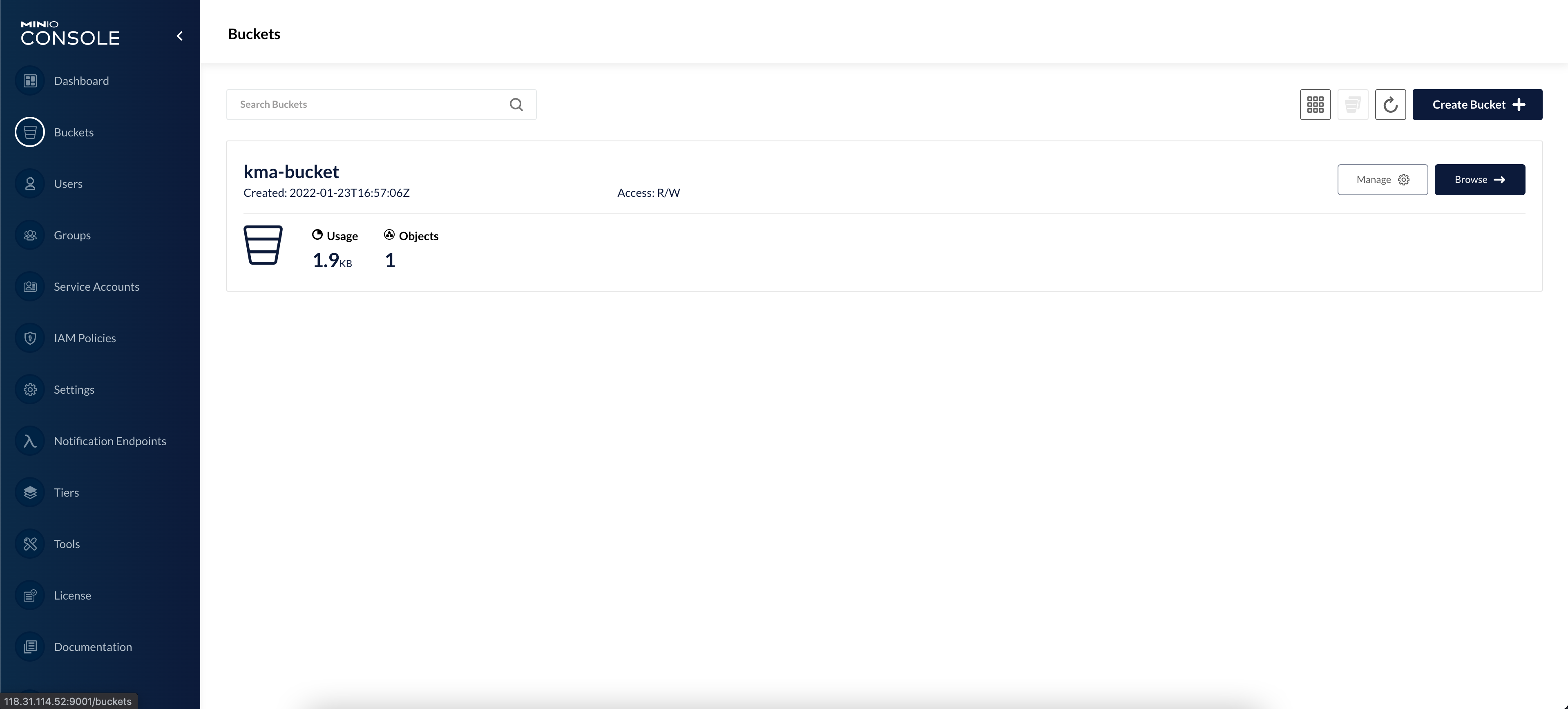
3.上传代码
配置文件
minio:
endpoint: http://118.31.114.52:9000/
accessKey: admin
secretKey: admin123321
bucketName: kma-bucket #存储桶
region: us-east-1 #默认(美国东区1),这也是亚马逊S3的默认区域 你可以通过MINIO_REGION 环境变量进行修改。如果不了解这块,建议不要随意修改
读取配置文件
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Minio {
private String endpoint;
private String accessKey;
private String secretKey;
private String bucketName;
private String region;
}
工具类
/**
* created with IntelliJ IDEA.
*
* @author: yz
* @date: 2022/1/23
* @time: 8:07 下午
* @description:
*/
@Slf4j
@AllArgsConstructor
public class MinioUtil {
private final MinioClient client;
/**
* 创建bucket
*
* @param bucketName bucket名称
*/
@SneakyThrows
public void createBucket(String bucketName) {
if (!client.bucketExists(bucketName)) {
client.makeBucket(bucketName);
}
}
/**
* 获取全部bucket
*/
@SneakyThrows
public List<Bucket> getAllBuckets() {
return client.listBuckets();
}
/**
* 根据bucketName获取信息
*
* @param bucketName bucket名称
*/
@SneakyThrows
public Optional<Bucket> getBucket(String bucketName) {
return client.listBuckets().stream().filter(b -> b.name().equals(bucketName)).findFirst();
}
/**
* 根据bucketName删除信息
*
* @param bucketName bucket名称
*/
@SneakyThrows
public void removeBucket(String bucketName) {
client.removeBucket(bucketName);
}
/**
* 获取文件外链
*
* @param bucketName bucket名称
* @param objectName 文件名称
* @param expires 过期时间 <=7
* @return url
*/
@SneakyThrows
public String getObjectURL(String bucketName, String objectName, Integer expires) {
return client.presignedGetObject(bucketName, objectName, expires);
}
/**
* 获取文件
*
* @param bucketName bucket名称
* @param objectName 文件名称
* @return 二进制流
*/
@SneakyThrows
public InputStream getObject(String bucketName, String objectName) {
return client.getObject(bucketName, objectName);
}
/**
* 获取文件信息
*
* @param bucketName bucket名称
* @param objectName 文件名称
* @throws Exception https://docs.minio.io/cn/java-client-api-reference.html#statObject
*/
public ObjectStat getObjectInfo(String bucketName, String objectName) throws Exception {
return client.statObject(bucketName, objectName);
}
/**
* 删除文件
*
* @param bucketName bucket名称
* @param objectName 文件名称
* @throws Exception https://docs.minio.io/cn/java-client-api-reference.html#removeObject
*/
public void removeObject(String bucketName, String objectName) throws Exception {
client.removeObject(bucketName, objectName);
}
/**
* 上传文件
*
* @param file 文件
* @param bucketName 存储桶
* @return
*/
public String uploadFile(MultipartFile file, String bucketName,String region,String fileName) throws Exception {
// 判断上传文件是否为空
if (null == file || 0 == file.getSize()) {
throw new KmaException(ResultEnum.UPLOAD_FILE_CANNOT_BE_EMPTY);
}
// 判断存储桶是否存在
createBucket(bucketName);
// 开始上传
BufferedInputStream bis = new BufferedInputStream(file.getInputStream());
client.putObject(PutObjectArgs.builder()
.bucket(bucketName)
.region(region)
.object(fileName)
.stream(bis, bis.available(), -1)
.contentType(file.getContentType())
.build());
return client.presignedGetObject(bucketName, fileName);
}
}
实现代码
/**
* created with IntelliJ IDEA.
*
* @author: yz
* @date: 2021/12/21
* @time: 9:36 下午
* @description:
*/
@RequiredArgsConstructor
public class MinioFileUploadServiceImpl implements FileUploadService {
private final StorageProperties properties;
@SneakyThrows
@Override
public String upload(MultipartFile file, String path, String fileName) {
MinioClient minioClient = new MinioClient(properties.getMinio().getEndpoint(), properties.getMinio().getAccessKey(), properties.getMinio().getSecretKey());
MinioUtil minioUtil = new MinioUtil(minioClient);
return minioUtil.uploadFile(file,properties.getMinio().getBucketName(),properties.getMinio().getRegion(),path+fileName);
}
@SneakyThrows
@Override
public void delete(String path) {
MinioClient minioClient = new MinioClient(properties.getMinio().getEndpoint(), properties.getMinio().getAccessKey(), properties.getMinio().getSecretKey());
MinioUtil minioUtil = new MinioUtil(minioClient);
minioUtil.removeObject(properties.getMinio().getBucketName(),path);
}
}
备注:
还有一种使用方式,后端生成MiniO的token,前端上传,更多操作查看文档
比如分片上传,mysql备份到MiniO